In the world of programming, there are many different tasks and algorithms that are commonly implemented to test a programmer’s understanding of certain concepts. One of these tasks is checking for Armstrong numbers. Before we dive into the actual programming part, it’s important to understand what an Armstrong number is.
What is an Armstrong Number?
An Armstrong number is a number that is equal to the sum of its own digits, each raised to the power of the number of digits. For example, let’s take 371. It’s a 3-digit number and the sum of the cubes of its digits (3^3 + 7^3 + 1^3) equals 371, which is the number itself. Thus, 371 is an Armstrong number.
Steps to Implement the Java Program
To implement a Java program that checks whether a number is an Armstrong number or not, the following steps can be taken:
- Input a number: First, the program needs to take a number as input from the user.
- Calculate the number of digits: This can be done by dividing the number by 10 in a loop until the number becomes 0.
- Calculate the sum of the power of digits: This involves taking each digit of the number, raising it to the power equal to the number of digits, and then adding it to the sum.
- Compare the sum and the original number: If the sum is equal to the original number, then the number is an Armstrong number, otherwise it is not.
Java Program to Check Armstrong Number
Now, let’s look at a simple Java program that checks if a given number is an Armstrong number or not.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 | import java.util.Scanner; public class CheckArmstrongNumber { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.println("Enter a number: "); int number = sc.nextInt(); int originalNumber, remainder, result = 0, n = 0; originalNumber = number; for (; originalNumber != 0; originalNumber /= 10, ++n); originalNumber = number; for (; originalNumber != 0; originalNumber /= 10) { remainder = originalNumber % 10; result += Math.pow(remainder, n); } if (result == number) System.out.println(number + " is an Armstrong number."); else System.out.println(number + " is not an Armstrong number."); } } |
How the Program Works
- The program takes a number as input from the user.
- It calculates the total number of digits in the number with the for loop. The loop runs until originalNumber becomes 0 and increments n each time, thus, n becomes the total number of digits.
- Then it calculates the sum of the power of the digits with another for loop. The loop runs until originalNumber becomes 0. Inside the loop, it calculates the remainder of originalNumber divided by 10, adds the power of the remainder (with n as the exponent) to result and then divides originalNumber by 10.
- Finally, it checks whether result is equal to the original number input by the user. If so, it prints that the number is an Armstrong number; otherwise, it prints that the number is not an Armstrong number.
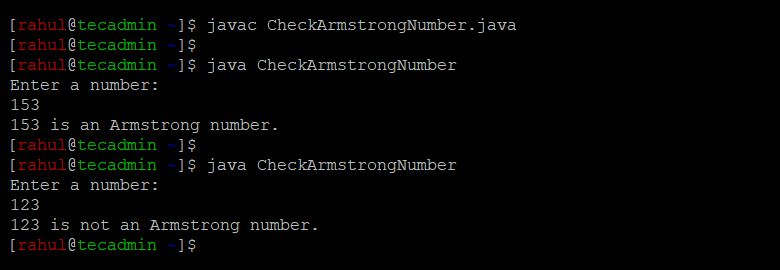
This program efficiently checks if a given number is an Armstrong number. Note that it works for both 3-digit numbers like 371, and numbers with more digits, like 9474 (since 9^4 + 4^4 + 7^4 + 4^4 = 9474). The application of such a program might seem limited, but the concepts used here are fundamental to many more complex operations in computer science, making this a great exercise for new learners.