Python is an easy-to-use programming language that many people use to do tasks automatically, like managing files and folders. It has built-in tools like “os” for handling files and “datetime” for working with dates and times. These tools make it simple to write scripts that can organize files and folders by using the current date and time. This makes it easier to manage large amounts of data.
In this guide, you will learn how to create files and folders with the current date and time in their names. This helps keep everything organized by date and time.
Setting Up Your Environment
Before working on programming, ensure that you have Python installed on your system. All the systems must have Python compiler installed to run applications. You can download it from the official Python website and install on your system freely. This tutorial will use Python 3, so make sure you have a version of Python 3.x installed.
Utilizing the datetime Module
The datetime module in Python is used for generating date and time based strings that can be used in naming files and folders. Here’s a simple example of how to use the datetime module to get the current date and time formatted as a string:
from datetime import datetime
# Get the current date and time
now = datetime.now()
# Format the date and time as a string
date_time_str = now.strftime("%Y-%m-%d_%H-%M-%S")
print(date_time_str)
This code snippet will print the current date and time in the format YYYY-MM-DD_HH-MM-SS, which is a common format for naming files and folders because it sorts chronologically when viewed in most file systems.
Creating Files and Folders with Date and Time Names
With the date and time string ready, you can now proceed to create files and folders using Python’s os module. Here’s how you can create a folder named with the current date and time:
import os
# Create a directory named after the current date and time
os.makedirs(date_time_str)
Similarly, you can create a file with a date and time-based name. If you want to create a text file, for example, you could append the .txt extension to the date_time_str variable:
# Create a text file with the current date and time as its name
file_name = f"{date_time_str}.txt"
with open(file_name, 'w') as file:
file.write("This is an example of creating a file with a date and time-based name.")
Craft a Complete Script to Create File and Folder
Let’s Craft a complete script that will create a directory with using current date. Also create a text file with current date and time in their name. This can be helpful for creating directory for daily backups and generate log files in a organized way.
The script is:
import os
from datetime import datetime
# Get the current date and time
now = datetime.now()
# Create a directory named after the current date and time
custom_dirname = now.strftime("%Y-%m-%d")
dir_name = f"Backup_{custom_dirname}"
os.makedirs(dir_name)
# Create a text file with the current date and time as its name
custom_filename = now.strftime("%Y-%m-%d_%H-%M-%S")
file_name = f"Log_{custom_filename}.txt"
with open(file_name, 'w') as file:
file.write("This is an sample log file with a date and time-based name.")
Save the above script in a file named “script.py” and execute it.
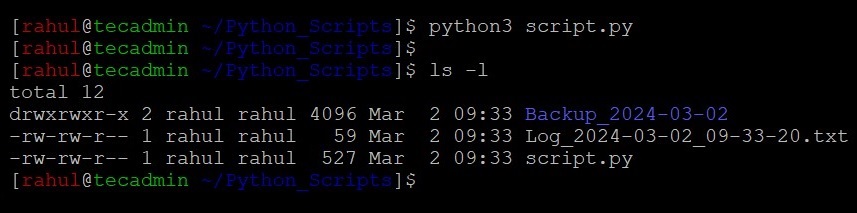
You will see a directory and a file created in current directory with date & time in there names.
Conclusion
Using Python to organize files can save time and help keep everything neat and tidy. By using Python’s built-in tools like “datetime” and “os,” you can make scripts that name files and folders with the current date and time. This makes it easier to manage files, work faster, and keep your computer organized.
Once you get the hang of these basic steps, you can try learning more advanced Python features and other tools to make your file management even better. Python gives you all the tools you need to create an easy and efficient system for managing your files.