In the world of programming, understanding how to manipulate and work with dates is fundamental. One common challenge is determining whether a given year is a leap year or not. Leap years, which occur roughly every four years, add an extra day to the calendar year, making February 29 days long instead of 28. This adjustment helps synchronize the calendar year with the astronomical year. In this tutorial, we will guide you through the process of writing an efficient C program to determine if a year is a leap year.
Understanding Leap Year Logic
Before diving into coding, it’s essential to understand the logic behind leap years. A year is considered a leap year if it is divisible by 4, except for end-of-century years, which must be divisible by 400. This means that the year 2000 was a leap year, while 1900 was not, despite both being divisible by 4. This rule corrects for the fact that a year is not exactly 365.25 days long.
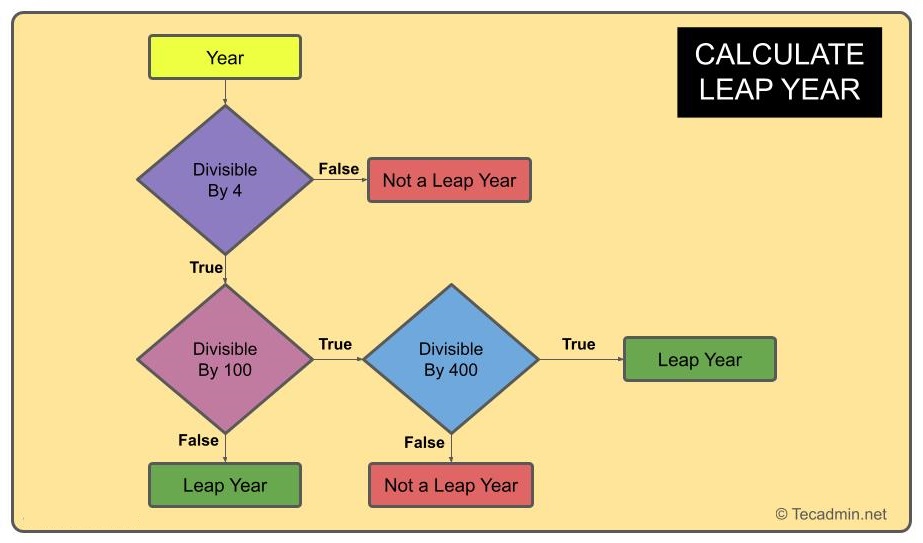
C Program to Check Leap Year
Open your text editor and start by including the standard input-output header file at the beginning of your program. Next, declare the main function where your program’s execution will begin:
#include <stdio.h>
// Main function where the program execution begins
int main() {
int year;
// Prompt the user to enter a year
printf("Enter a year: ");
scanf("%d", &year);
// Check if the year is a leap year
if ((year % 4 == 0 && year % 100 != 0) || (year % 400 == 0)) {
printf("%d is a leap year.\n", year);
} else {
printf("%d is not a leap year.\n", year);
}
return 0;
}
This code checks if the year is divisible by 4 but not by 100, or if it’s divisible by 400. If either condition is true, the program prints that the year is a leap year. Otherwise, it states that the year is not a leap year.
Compiling and Running Your Program
To run this program, you can either use a online C compiler or a software application.
Also you can use command line C compiler. After saving your program, open a terminal or command prompt, navigate to the directory containing your program, and compile it using the GCC compiler:
gcc -o leapyear leapyear.c
This command compiles your leapyear.c file and creates an executable named leapyear. To run your program, simply type:
./leapyear
Enter a year when prompted, and your program will tell you whether it’s a leap year.
Conclusion
Congratulations! You have successfully written an efficient C program to determine leap years. This tutorial not only taught you how to identify leap years but also provided a practical application of conditional statements in C. With this knowledge, you can now tackle more complex date-related challenges in your programming projects.
Understanding the logic behind leap years and implementing it in C sharpens your programming skills and deepens your knowledge of how software can interact with time and calendars. Keep experimenting with different approaches and optimizations to enhance your programming proficiency.