Creating files and folders with names based on the current date and time is a crucial aspect of organizing data, automating backups, and logging in Windows environments. Windows Batch Script, the scripting language for Windows Command Prompt, offers straightforward methods to incorporate date and time stamps into your filenames and directory names. This article explores how to achieve this, ensuring your scripts can dynamically generate unique and descriptive names.
Understanding Windows Batch Script Date & Time
In Windows Batch Script, the %DATE% and %TIME% environment variables provide the current date and time, respectively. However, their format is influenced by the system’s regional settings, which can vary. Typically, %DATE% returns the date in the format “Fri 03/01/2024“, and %TIME% provides the time in the format “12:30:45.78”.
Formatting Date and Time
To create file or folder names with date and time, you must extract and format these values accordingly. Since direct formatting options like in Linux’s date command are not available, you’ll often need to use substring extraction and replacement techniques.
Extracting Date Components
Here’s how you can extract year, month, and day from the %DATE% variable, assuming the format is MM/DD/YYYY:
set YEAR=%DATE:~10,4%
set MONTH=%DATE:~4,2%
set DAY=%DATE:~7,2%
Extracting Time Components
Similarly, to extract hour, minute, and second from %TIME%, you might use:
set HOUR=%TIME:~0,2%
set MINUTE=%TIME:~3,2%
set SECOND=%TIME:~6,2%
Generating Names Based on Date and Time
With the date and time components extracted, you can now combine them to form unique file or folder names.
Creating a Timestamped File Name
set FILENAME=Log_%YEAR%-%MONTH%-%DAY%_%HOUR%-%MINUTE%-%SECOND%.txt
echo Log entry > %FILENAME%
This script creates a log file named like Log_2024-03-01_12-30-45.txt.
Creating a Directory Based on the Date
For organizing backups or logs by date, you might create a directory like so:
set DIRNAME=Backup_%YEAR%-%MONTH%-%DAY%
mkdir %DIRNAME%
This command creates a directory named Backup_2024-03-01.
Handling Single-Digit Day and Month
One challenge is that single-digit months and days can lead to names with unexpected formats due to leading spaces. To ensure a consistent two-digit format, you can add a zero padding where necessary:
if %MONTH% LSS 10 set MONTH=0%MONTH:~1,1%
if %DAY% LSS 10 set DAY=0%DAY:~1,1%
A Sample Batch Script with Date & Time
To utilize the script in a Windows environment for generating timestamped filenames and directory names, follow these steps: Create a new file named script.bat and input the content outlined below. This script is designed to extract the current date and time, format these elements for consistency, and then employ them to construct a unique log file and a backup directory.
@echo off
:: Extract year, month, and day from the system's date
set YEAR=%DATE:~10,4%
set MONTH=%DATE:~4,2%
set DAY=%DATE:~7,2%
:: Ensure month and day are two digits (add leading zero if necessary)
if %MONTH% LSS 10 set MONTH=0%MONTH:~1,1%
if %DAY% LSS 10 set DAY=0%DAY:~1,1%
:: Extract hour, minute, and second from the system's time
set HOUR=%TIME:~0,2%
set MINUTE=%TIME:~3,2%
set SECOND=%TIME:~6,2%
:: Ensure hour, minute, and second are two digits (add leading zero if necessary)
if %HOUR% LSS 10 set HOUR=0%HOUR:~1,1%
if %MINUTE% LSS 10 set MINUTE=0%MINUTE:~1,1%
if %SECOND% LSS 10 set SECOND=0%SECOND:~1,1%
:: Create a log file with the current timestamp in its name
set FILENAME=Log_%YEAR%-%MONTH%-%DAY%_%HOUR%-%MINUTE%-%SECOND%.txt
echo Log entry > %FILENAME%
:: Create a directory with the current timestamp in its name
set DIRNAME=Backup_%YEAR%-%MONTH%-%DAY%
mkdir %DIRNAME%
After saving the script as script.bat, you can run it by double-clicking the file or executing it from the command prompt.
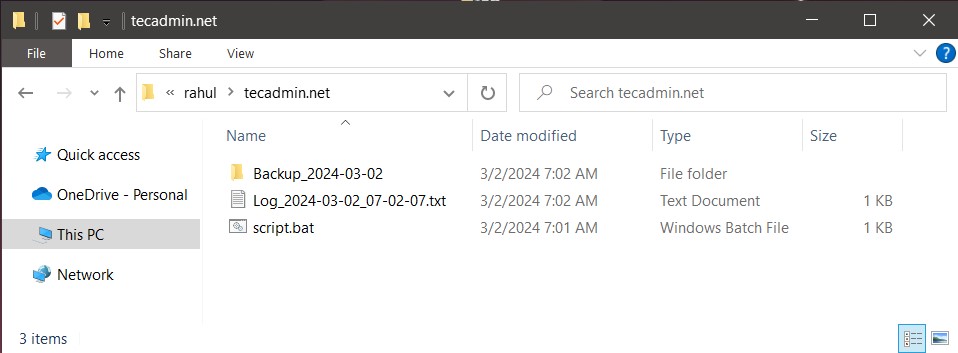
You will find that a directory is created with the name “Backup_2024-03-02”. Also, a file created in the current directory with the name “Log_2024-03-02_07-02-07.txt” (Filename will be according to current date and time and will change during your testing)
Conclusion
Generating file and folder names based on the current date and time in Windows Batch Script requires a bit more manual effort compared to other scripting environments. However, by extracting and formatting date and time components, you can create meaningful, unique names for files and directories. This approach is invaluable for automated data management tasks, ensuring your scripts can handle files in an organized, chronological manner.
27 Comments
This script with modified date vs current date is just what I need.
How about 12-hour only?
Thank you so much for sharing your script with us.
I am using it to generate differential backups using the command-line version of 7-ZIP.
How to make a folder in a specified directory. I used a task scheduler to automate the folder creation, however, it is creating the folder in C:/Windows.
Hi Gaurav, You can add the folder location as prefix to mkdir command:
mkdir D:\\parrent-dir\\%SUBFILENAME%
Doesn’t work at all for me as posted. Mainly because my date variable is in the format 17/05/2022 . No day of week, and (unlike those weird Americans, who are just awkward for the sake of it – what’s next, time expressed as mm:hh:ss ? ) we have our dates as DD/MM/YYYY in the UK.
Windows formats dates differently for everyone, depending on location settings etc. Another top tip is to use an example date with DD over 12 (instead of 2nd November try 21st November), so we can clearly see which bit is the month and which the days.
Maybe you should suggest readers echo their %date% variable to start with, so they can find out what it looks like. A little knowledge is a dangerous thing.
Excellent, Very useful
If you want the file to be inside the directory you created then the last line needs to be
echo “Welcome Here!”>%fecha1%\access_%fecha1%.log
There is no need for the line
SET fechaTIME1=%time:~0,2%%time:~3,2%%time:~6,2%
That last example works well apart from the quotation marks around Welcome Here! create odd characters so I removed them and if you intended for the text file to go in the folder created than you need to change the last line to
echo “Welcome Here!”>%fecha1%\access_%fecha1%.log
I’m having a hard time figuring out how a Date/Time like Nov 02, 2017 15:41:36 can come up with a file name like 20170306-143822
Hi Rick, Thanks for pointing out. I have corrected the article.
Rahul Kumar:-
Thanks for sharing this.
It is a very clean and precise code snippet for getting the current timestamp in a DOS Batch file.
Daniel Adeniji
Works Great – Thanks
oh, I used your environment variables to make a Win 10 filename, the last line just echos command line to test! Thanks again!
echo off
set CUR_YYYY=%date:~10,4%
set CUR_MM=%date:~4,2%
set CUR_DD=%date:~7,2%
set CUR_HH=%time:~0,2%
if %CUR_HH% lss 10 (set CUR_HH=0%time:~1,1%)
set CUR_NN=%time:~3,2%
set CUR_SS=%time:~6,2%
set CUR_MS=%time:~9,2%
set SUBFILENAME=%CUR_YYYY%%CUR_MM%%CUR_DD%-%CUR_HH%%CUR_NN%%CUR_SS%
echo “ffmpeg -f image2 -framerate 15 -i SC-%%07d.jpg -s 640×360 SC-Movie-%SUBFILENAME%.avi”
Great, but your date script doesn’t work (win 10).
I used:
set ToDaysDate=%date:~6,4%%date:~3,2%%date:~0,2%
set CUR_NN=%time:~3,2%
set CUR_SS=%time:~6,2%
set CUR_MS=%time:~9,2%
set SUBFILENAME=%ToDaysDate%-%CUR_HH%%CUR_NN%%CUR_SS%
It’s a common mistake to make. You reference %CUR_HH% but you haven’t initialised it yet. Initialise it as follows:
set CUR_HH=%time:~0,2%
if %CUR_HH% lss 10 (set CUR_HH=0%time:~1,1%)
Thank you! It works great for me!
Thanks so much for this, tested on win10 pro and it works perfectly.
Windows 10 as follows:
set CUR_YYYY=%date:~6,4%
set CUR_MM=%date:~3,2%
set CUR_DD=%date:~0,2%
set CUR_HH=%time:~0,2%
if %CUR_HH% lss 10 (set CUR_HH=0%time:~1,1%)
set CUR_NN=%time:~3,2%
set CUR_SS=%time:~6,2%
set CUR_MS=%time:~9,2%
set SUBFILENAME=%CUR_YYYY%%CUR_MM%%CUR_DD%-%CUR_HH%%CUR_NN%%CUR_SS%
mkdir %SUBFILENAME%
cd %SUBFILENAME%
echo “Welcome Here!” > access_%SUBFILENAME%.log
The string pointers for the Day and the Month are wrong
it should be
set CUR_MM=%date:~7,2%
set CUR_DD=%date:~4,2%
The solution above breaks if you change locale. See https://serverfault.com/questions/227345/locale-unaware-date-and-time-in-batch-files
how to generate the day of the week?
Thank you very much, was very helpful, BUT:
– you should mention that the date and time string is depending on the format at the local settings of windows.
– people should adjust the extraction of the string, as extra help:
set D=1234567890
set A=%D:~4,2% gives ’56’ , so 2 char AFTER the 4.
-it is better to first save the string to a variable instead of using the %date% and %time% again.
for example: if I use your code on Nov 30, 2017 on 23:59, say 0,002sec before midnight, the last lines could be executed on the next day: 20171101 , so you miss a month!
More a problem with the time, when you want precision 03,98sec might become SS 03 + MS 05 = 03,05 in stead of the expected 03,98 or 4,05
So start with :
set D=%date%
and change to :
set CUR_MM=%D:~4,2%
Just my 2 cents, hope it helps.
SCS
Hello Rahull,
this script is fine, work for me, but: now is date 07.01.2019 today, and %CUR_DD% is 01 instead 07. Why? What is wrong?
I testing on Win 10.
windows 10 -> sysntax error
SET fechaTIME=%time:~0,2%:%time:~3,2%:%time:~6,2%
SET fechaTIME1=%time:~0,2%%time:~3,2%%time:~6,2%
SET fechaDATE=%date:~6,4%%date:~3,2%%date:~0,2%
SET fecha1=%fechaDATE%-%fechaTIME1%
echo on
mkdir %fecha1%
echo “Welcome Here!”>access_%fecha1%.log