Python is a high-level programming language that is widely used for automating repetitive tasks, including file and directory management. Its standard library provides modules such as os and datetime that simplify file operations and manipulation of dates and times, respectively. This capability allows users to create scripts that can automate the organization of files and directories based on the current date and time, making the management of large volumes of data more manageable and efficient.
This article will guide you through the process of automating file management with Python, focusing on crafting date and time-based file and folder names.
Setting Up Your Environment
Before diving into the code, ensure that you have Python installed on your system. You can download it from the official Python website. This tutorial will use Python 3, so make sure you have a version of Python 3.x installed.
Utilizing the datetime Module
The datetime module in Python is crucial for generating date and time strings that can be used in naming files and folders. Here’s a simple example of how to use the datetime module to get the current date and time formatted as a string:
from datetime import datetime
# Get the current date and time
now = datetime.now()
# Format the date and time as a string
date_time_str = now.strftime("%Y-%m-%d_%H-%M-%S")
print(date_time_str)
This code snippet will print the current date and time in the format YYYY-MM-DD_HH-MM-SS, which is a common format for naming files and folders because it sorts chronologically when viewed in most file systems.
Creating Files and Folders with Date and Time Names
With the date and time string ready, you can now proceed to create files and folders using Python’s os module. Here’s how you can create a folder named with the current date and time:
import os
# Create a directory named after the current date and time
os.makedirs(date_time_str)
Similarly, you can create a file with a date and time-based name. If you want to create a text file, for example, you could append the .txt extension to the date_time_str variable:
# Create a text file with the current date and time as its name
file_name = f"{date_time_str}.txt"
with open(file_name, 'w') as file:
file.write("This is an example of creating a file with a date and time-based name.")
Craft a Complete Script to Create File and Folder
Let’s Craft a complete script that will create a directory with using current date. Also create a text file with current date and time in their name. This can be helpful for creating directory for daily backups and generate log files in a organized way.
The script is:
import os
from datetime import datetime
# Get the current date and time
now = datetime.now()
# Create a directory named after the current date and time
custom_dirname = now.strftime("%Y-%m-%d")
dir_name = f"Backup_{custom_dirname}"
os.makedirs(dir_name)
# Create a text file with the current date and time as its name
custom_filename = now.strftime("%Y-%m-%d_%H-%M-%S")
file_name = f"Log_{custom_filename}.txt"
with open(file_name, 'w') as file:
file.write("This is an sample log file with a date and time-based name.")
Save the above script in a file named “script.py” and execute it.
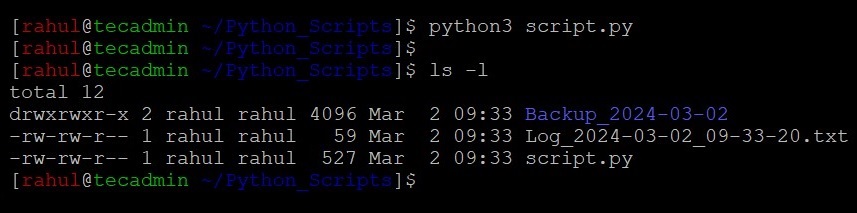
You will see a directory and a file created in current directory with date & time in there names.
Conclusion
Automating file management with Python not only saves time but also introduces a level of precision and order to file organization that is hard to achieve manually. By leveraging Python’s datetime and os modules, you can easily create scripts that name files and folders based on the current date and time, making it easier to manage data, improve productivity, and maintain a clean digital workspace.
As you become more comfortable with these basic operations, consider exploring more advanced Python features and third-party modules to further enhance your file management scripts. With Python, the tools to create a highly efficient, automated file management system are at your fingertips.