Creating file and folder names based on the current date and time is a common requirement in scripting and automation tasks. PowerShell, a powerful scripting language and shell developed by Microsoft, provides a flexible and easy way to accomplish this. This capability is particularly useful for log files, backups, and any scenario where unique, time-stamped file names are necessary. In this article, we’ll explore how to use PowerShell to generate date and time-based names for files and folders.
Getting the Current Date and Time
In PowerShell, you can get the current date and time by using the Get-Date cmdlet. This cmdlet is highly customizable and can be formatted to suit your needs.
Get-Date
Formatting the Date and Time
To format the date and time in a specific way, you can use the -Format parameter of the Get-Date cmdlet. PowerShell supports custom date and time formats, allowing you to create strings suitable for file and folder names.
Get-Date -Format "yyyy-MM-dd_HH-mm-ss"
This command will produce a string formatted as 2024-03-01_12-30-45, which is a common format for timestamping files.
Creating a File with a Timestamp
To create a file with a name based on the current date and time, you can combine Get-Date with the New-Item cmdlet.
$timestamp = Get-Date -Format "yyyy-MM-dd_HH-mm-ss"
New-Item -Path "C:\Logs\Log_$timestamp.txt" -ItemType "file"
This script creates a new log file with the current date and time as part of its name in the C:\Logs directory.
Creating a Folder with a Timestamp
Similarly, you can create a directory (folder) with a timestamp in its name by slightly modifying the above script.
$timestamp = Get-Date -Format "yyyy-MM-dd_HH-mm-ss"
New-Item -Path "C:\Backups\Backup_$timestamp" -ItemType "directory"
This creates a new backup folder in the C:\Backups directory, using the current date and time as part of the folder name.
Advanced Formatting Options
PowerShell’s Get-Date -Format supports a wide range of format specifiers, allowing you to customize the date and time format to your exact requirements. For example, if you only need the date for a daily log file, you can format the date without the time.
Get-Date -Format "yyyy-MM-dd"
A Sample PowerShell Script to Create Files and Folders with Date & Time
Here is a sample PowerShell script that will create file and folder with date and time in there names.
# Get the current date and time
$CurrentDate = Get-Date
# Format date and time components
$Year = $CurrentDate.ToString("yyyy")
$Month = $CurrentDate.ToString("MM")
$Day = $CurrentDate.ToString("dd")
$Hour = $CurrentDate.ToString("HH")
$Minute = $CurrentDate.ToString("mm")
$Second = $CurrentDate.ToString("ss")
# Combine date and time components to form a filename
$Filename = "Log_$Year-$Month-$Day" + "_$Hour-$Minute-$Second.txt"
# Create a log file with a timestamp in its name
"Log entry" | Out-File $Filename
# Combine date components to form a directory name for backups
$DirName = "Backup_$Year-$Month-$Day"
# Create a backup directory with a timestamp in its name
New-Item -ItemType Directory -Path $DirName
Save the above script in a file named script.ps1 and execute it.
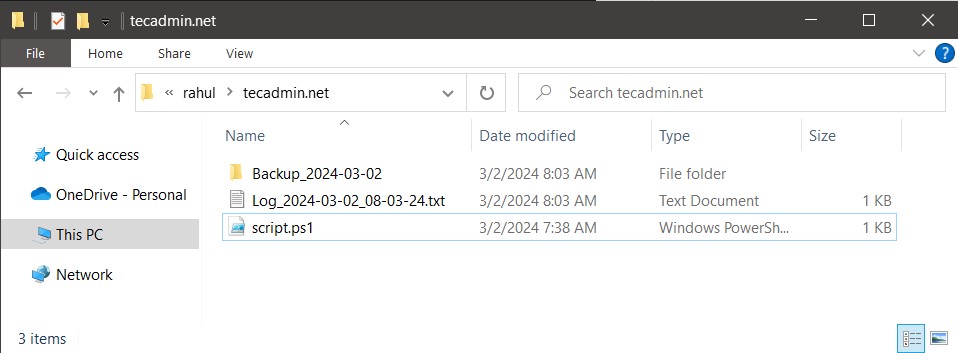
The script will A log file named in the format Log_YYYY-MM-DD_HH-MM-SS.txt will be created. This file will contain the text “Log entry”. Also it will create a directory named in the format Backup_YYYY-MM-DD will also be created in the current working directory.
Conclusion
PowerShell offers a powerful and flexible way to work with date and time, making it easy to include timestamps in file and folder names. This is invaluable for creating easily sortable, unique names for logs, backups, and other files that are created as part of automated processes. By mastering the use of the Get-Date cmdlet and its formatting options, you can enhance your PowerShell scripts to handle files and directories more effectively.