JavaScript, as one of the most popular programming languages worldwide, offers a plethora of possibilities. Among those possibilities is the option to detect the status of the Caps Lock key. This detection can be quite important in various scenarios, such as when dealing with password inputs where the user might not realize that their Caps Lock key is on. In this article, we will take a deep dive into how you can detect whether the Caps Lock key is turned on or off in JavaScript.
What you will learn?
- Understanding the KeyboardEvent object in JavaScript
- Detecting the status of the Caps Lock key
The KeyboardEvent Object
To understand how to detect the Caps Lock status, we must first understand the KeyboardEvent object. Whenever a user interacts with the keyboard, such as when pressing or releasing a key, a KeyboardEvent is fired. This event carries useful information about the key pressed, whether any ‘modifier’ keys like Shift or Ctrl are pressed, and more.
Caps Lock Detection
To detect the status of the Caps Lock key, we can use the getModifierState() method that is available on the KeyboardEvent object. This method returns a Boolean value that indicates whether a modifier key such as Caps Lock is active. The method accepts one argument, which is a string representing the modifier key.
Here’s a basic example of using getModifierState() to detect the Caps Lock status:
1 2 3 4 | document.addEventListener('keydown', function(event) { var capsIsOn = event.getModifierState('CapsLock'); console.log('Caps Lock status: ', capsIsOn ? 'On' : 'Off'); }); |
In the example above, we’re attaching an event listener to the document that listens for the ‘keydown’ event. The callback function receives the event object. We then call `event.getModifierState(‘CapsLock’)`, which will return true if the Caps Lock key is active and false otherwise.
Implementing Visual Indicators for Caps Lock
Detecting Caps Lock status is most beneficial when implemented with real-time user feedback, such as during password input. Let’s create a function that shows a warning message when Caps Lock is on:
1 2 3 4 5 6 7 8 9 10 | document.getElementById('password').addEventListener('keyup', function(event) { var capsLockOn = event.getModifierState('CapsLock'); var warningElement = document.getElementById('caps-warning'); if(capsLockOn) { warningElement.style.display = 'block'; } else { warningElement.style.display = 'none'; } }); |
In this code, we have an input field with the ID ‘password’, and a warning message with the ID ‘caps-warning’. When the Caps Lock key is on, the warning message is displayed. When the Caps Lock key is off, the warning message is hidden.
Create an HTML Page
Here’s an HTML demo that includes an input field for a password and a warning message that appears when the Caps Lock key is turned on. You can also view this demo here:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 | <!DOCTYPE html> <html> <head> <title>Detect Caps Lock in JavaScript</title> <style> #caps-warning { display: none; color: red; } </style> </head> <body> <h1>Caps Lock Detection in JavaScript</h1> <form> <label for="password">Enter your password:</label><br> <input type="password" id="password" name="password"><br> <p id="caps-warning">Warning: Caps Lock is on!</p> </form> <script> document.getElementById('password').addEventListener('keyup', function(event) { var capsLockOn = event.getModifierState('CapsLock'); var warningElement = document.getElementById('caps-warning'); if(capsLockOn) { warningElement.style.display = 'block'; } else { warningElement.style.display = 'none'; } }); </script> </body> </html> |
In this demo, the warning message is hidden by default using CSS (display: none). The script listens for the ‘keyup’ event on the password input field, and if the Caps Lock is on, it changes the CSS of the warning message to `display: block`, showing the warning message. If the Caps Lock is turned off, it hides the warning message again by setting `display: none`.
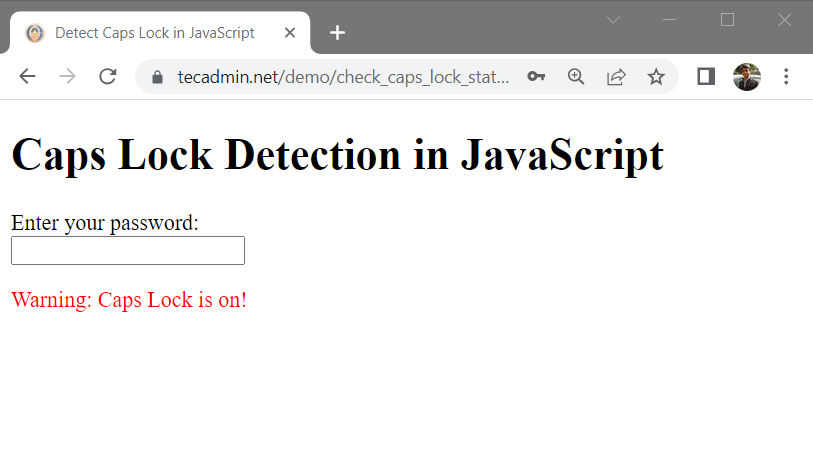
Points to Remember
This approach only detects whether the Caps Lock key is on at the time of the key press. It does not continually monitor the status of the key.
The Caps Lock status can vary between different devices and operating systems. Always ensure your implementation works across different environments.
Conclusion
Detecting the status of the Caps Lock key in JavaScript might seem complex at first, but once you understand the KeyboardEvent object and its methods, it becomes an easy task. Implementing such features can significantly enhance user experience by providing helpful feedback when required. As always, it’s vital to test your implementation on different devices and operating systems to ensure compatibility. Happy coding!