JavaScript Object Notation, or JSON, stands as a highly efficient format for data interchange. Primarily designed for data storage and transmission, it originates from JavaScript but has evolved to be independent of programming language. Its creation aimed at introducing a text-based format that is both human-readable and easy to use.
This guide will explore handling JSON in Python, utilizing the built-in JSON package to manipulate JSON structures.
1. Parsing JSON to Python Dictionary
The json.loads()
function is essential for converting a JSON string into a Python dictionary, requiring the JSON data to be passed as a string.
Consider the following example where employee represents a JSON string, and employee_dict is the resulting Python dictionary:
import json
employee = '{"First_Name": "John", "Second_Name": "Doe", "id": "01", "Department": "Health"}'
employee_dict = json.loads(employee)
print(employee_dict)
Output:
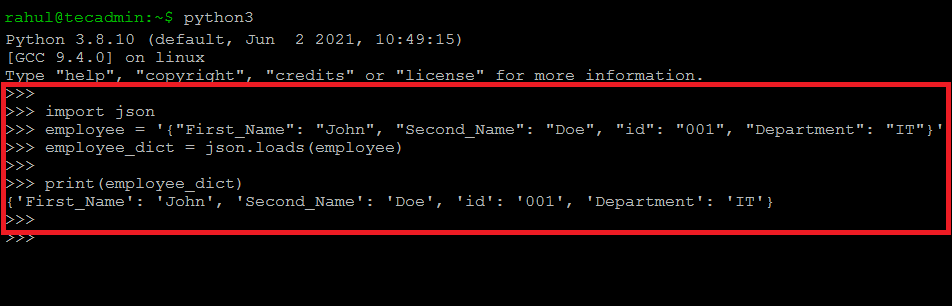
2. Converting Python Dictionary to JSON String
After learning to parse JSON into a Python dictionary, the next step is converting a Python dictionary back into a JSON string using json.dumps()
.
Example:
import json
employee_dict = {'First_Name': 'John', 'Second_Name': 'Doe', 'id': '01', 'Department': 'Health'}
employee = json.dumps(employee_dict)
print(employee)
Output:
3. Reading a JSON File
To read a JSON file in Python, json.load()
is used, as shown in the following steps:
- Create a `data.json` file with the desired content.
- Utilize the script below to read the JSON file and output its content.
import json with open('/home/rahul/data.json') as f: employee_data= json.load(f) print(employee_data)
Output:
4. Writing JSON Data to a File
Python’s json.dump()
method enables writing a Python dictionary as a JSON string to a file, as demonstrated below:
import json
employee_dict = {"First_Name": "John", "Second_Name": "Doe", "id": "01", "Department": "Health"}
with open('employee.json', 'w') as json_file:
json.dump(employee_dict, json_file)
Output:
Conclusion
JSON’s versatility and simplicity have cemented its status as a preferred method for data storage and transfer among developers. This overview provided insights into essential JSON operations in Python, covering how to parse, generate, read, and write JSON data effectively.