Kubernetes has become the go-to solution for container orchestration, allowing developers to deploy, manage, and scale containerized applications with ease. However, setting up a Kubernetes cluster can be a complex process, involving numerous steps and configurations. This is where Ansible, an open-source automation tool, comes into play. By automating the deployment process, Ansible can significantly simplify the creation of a Kubernetes cluster.
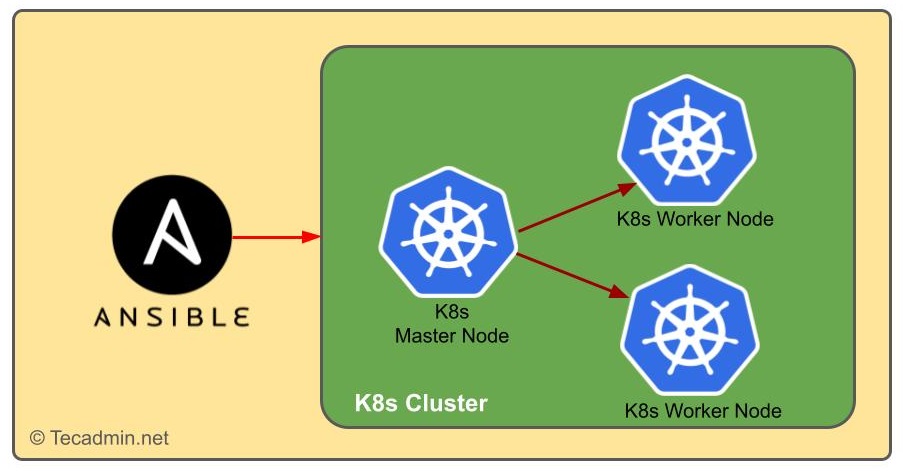
In this guide, we’ll walk you through the process of building a Kubernetes cluster using Ansible. We assumes that all nodes in K8s cluster is running with Ubuntu Linux operating system.
Prerequisites
Before we dive into the setup process, ensure that you have the following prerequisites in place:
- Ansible: You need to have Ansible installed on your control machine. The control machine is where you’ll run the Ansible scripts, and it can be your local machine or a server.
- Target Nodes: These are the machines where Kubernetes will be installed. You’ll need at least two nodes: one for the master node and one for a worker node. Ensure these nodes are running a compatible Linux distribution.
- SSH Access: Ansible communicates with the target nodes over SSH. You must have SSH access configured for all the target nodes from your control machine.
Step 1: Ansible Installation
To begin, set up Ansible on your control machine. The process involves installing Ansible, which varies depending on your operating system. Use the following commands based on your distribution:
sudo apt-get update
sudo apt-get install ansible
Step 2: Setup Inventory File
After installing Ansible, proceed to configure the inventory file. Create a file named hosts.ini in your working directory, which will enumerate all target nodes along with their respective IP addresses. To enhance clarity, organize them into groups such as masters and workers. Here’s an example:
[masters]
master ansible_host=192.168.1.100
[workers]
worker1 ansible_host=192.168.1.101
worker2 ansible_host=192.168.1.102
Step 3: Setup K8s Cluster Playbook
This playbook will help you with setting up a Kubernetes cluster using Ubuntu systems. It includes tasks for disabling the firewall, turning off swap, configuring network settings, adding the Docker repository, installing Docker and its components, adjusting container runtime settings, adding the Kubernetes repository, and installing Kubernetes components (kubelet, kubeadm, kubectl). Notably, kubectl is only installed on nodes identified as masters. The playbook demonstrates a comprehensive approach to preparing systems for Kubernetes, focusing on required services, security configurations, and essential Kubernetes components.
Create a setup-playbook.yaml file and add the below content:
- name: Initialize master and worker nodes
hosts: all
tasks:
- name: disable UFW firewall for labs
service:
name: ufw
state: stopped
enabled: false
- name: Disable SWAP
shell: |
swapoff -a
- name: Disable SWAP in fstab
lineinfile:
path: /etc/fstab
regexp: '^.*swap.*$'
line: '#\0'
backrefs: yes
- name: ensure net.bridge.bridge-nf-call-ip6tables is set to 1
sysctl:
name: net.bridge.bridge-nf-call-iptables
value: '1'
state: present
reload: yes
- name: Installation of apt-utils
apt:
name: apt-transport-https
state: present
update_cache: yes
- name: Adding Docker GPG key
ansible.builtin.apt_key:
url: https://download.docker.com/linux/ubuntu/gpg
state: present
- name: Adding Docker Repository
apt_repository:
repo: deb [arch=amd64] https://download.docker.com/linux/ubuntu {{ ansible_distribution_release }} stable
state: present
- name: Installation of Docker
apt:
name: "{{ item }}"
state: present
loop:
- docker-ce
- docker-ce-cli
- containerd.io
- docker-compose
- name: Setting value of SystemdCgroup
shell: |
containerd config default | sudo tee /etc/containerd/config.toml | grep SystemdCgroup
sed -i 's/SystemdCgroup = false/SystemdCgroup = true/g' /etc/containerd/config.toml
- name : Starting Service of Docker
service:
name: docker
state: started
enabled: yes
- name: Add Kubernetes apt repository
apt_repository:
repo: deb https://apt.kubernetes.io/ kubernetes-xenial main
state: present
- name: Install kubelet and kubeadm
apt:
name: "{{ item }}"
state: present
loop:
- kubeadm
- kubelet
- name: start kubelet
service:
name: kubelet
enabled: yes
state: started
- name: install kubectl
apt:
name: kubectl
state: present
when: "'masters' in group_names"
Execute the playbook by running:
ansible-playbook -i hosts.ini setup-playbook.yml
Step 4: Setup Master Node
This Ansible playbook is designed for configuring the master node of a Kubernetes cluster. It initializes the Kubernetes cluster with specific network configurations, creates a .kube directory, and copies the kube config file to the user’s home directory for cluster management. It also installs the Calico network plugin to handle pod networking, ensuring pods can communicate with each other across nodes. The playbook automates the initial setup process, simplifying the deployment of a Kubernetes cluster.
Create a file named master-playbook.yml and add below content:
- name: Configuration of master node
hosts: masters
tasks:
- name: initialize K8S cluster
shell: kubeadm init --pod-network-cidr=172.16.0.0/16 --apiserver-advertise-address=192.168.100.5 --ignore-preflight-errors=all
- name: create .kube directoryi and copy kube config file
shell: "{{ item }}"
loop:
- mkdir -p $HOME/.kube
- cp -i /etc/kubernetes/admin.conf $HOME/.kube/config
- chown $(id -u):$(id -g) $HOME/.kube/config
- name: install Pod network
become: yes
shell: kubectl apply -f https://raw.githubusercontent.com/projectcalico/calico/v3.25.0/manifests/calico.yaml >> pod_network_setup.txt
args:
chdir: $HOME
creates: pod_network_setup.txt
Execute the playbook with command:
ansible-playbook -i hosts.ini master-playbook.yml
Step 5: Setup Worker Nodes Playbook
This playbook is used for automating the Kubernetes node joining process. It executes on all nodes, generating a join command on the master node and distributing it to worker nodes. Initially, it fetches the join command using kubeadm token create and stores it. Then, it sets a fact with this command for use on worker nodes. Finally, worker nodes use this command to join the Kubernetes cluster, bypassing preflight checks. This playbook streamlines the cluster expansion process by automating the node joining procedure.
Create a file named worker-playbook.yml and add below content:
- name: Generating token on master node and token deployment to worker node
hosts: all
gather_facts: false
tasks:
- name: get join command
shell: kubeadm token create --print-join-command
register: join_command_raw
when: "'masters' in group_names"
- name: set join command
set_fact:
join_command: "{{ join_command_raw.stdout_lines[0] }}"
when: "'masters' in group_names"
- name: join cluster
shell: "{{ hostvars['master'].join_command }} --ignore-preflight-errors all >> node_joined.txt"
args:
chdir: $HOME
creates: node_joined.txt
when: "'workers' in group_names"
Execute the playbook with command:
ansible-playbook -i hosts.ini worker-playbook.yml
Step 5: Verifying the Cluster
Once the playbooks have been executed, you can verify that the Kubernetes cluster is set up correctly.
On the master node, run:
kubectl get nodes
You should see all the nodes listed with their statuses as Ready.
Conclusion
By following these steps, you have successfully set up a Kubernetes cluster using Ansible. This method offers a scalable and repeatable process, making it easier to manage and automate the deployment of Kubernetes clusters. As you become more familiar with Ansible and Kubernetes, you can further customize your playbooks to suit your specific requirements, such as adding more worker nodes or configuring advanced features. Happy orchestrating!