Imagine you have a collection of boxes, and inside each box, there is a small note. The note inside the box either tells you where the next box is or tells you there’s no more box left. This is, in a very simplified way, the concept of a singly linked list. Let’s dive into how you can create one in the C programming language.
What is a Singly Linked List?
In more technical terms, a singly linked list is a collection of nodes where each node has two components:
- Data – The actual information you want to store (this could be a number, a character, or any kind of data).
- Next – A pointer to the next node in the sequence (or NULL if there’s no subsequent node).
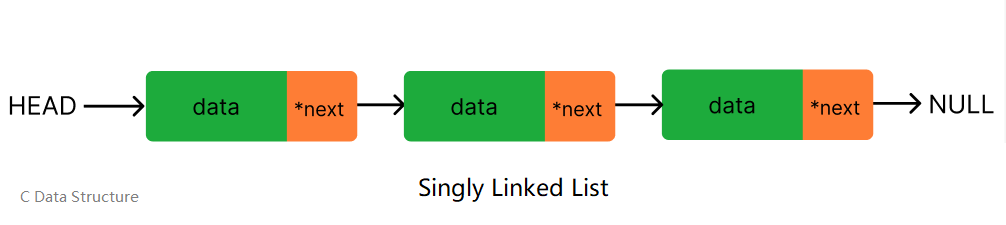
Setting Up the Singly Linked-list
To start, we need to define the structure of our node:
typedef struct Node {
int data; // the data part
struct Node* next; // the pointer part, pointing to the next node
} Node;
Here, the typedef keyword is used to give a name (Node) to our structure, making it easier to use later.
Functions to Work with Our Singly Linked List
Next, we will discuss functions about working with linked list, where we will create a linked list, add new element and display the linked list.
- Creating a New Node: This function will help us create a new node and return its address.
Node* createNewNode(int data) { Node* newNode = (Node*)malloc(sizeof(Node)); // Allocating memory for the new node if(!newNode) { // Check if memory allocation was successful printf("Memory allocation failed\n"); return NULL; } newNode->data = data; // Setting the data newNode->next = NULL; // Initially, the next pointer is set to NULL return newNode; // Returning the new node's address }
- Appending Data to the End: This function will help us add a new node at the end of our linked list.
void append(Node** head, int data) { Node* newNode = createNewNode(data); // Create a new node if(*head == NULL) { // If the list is empty *head = newNode; // Make this new node the first node return; } Node* lastNode = *head; // Start from the first node while(lastNode->next) { // Traverse till the last node lastNode = lastNode->next; } lastNode->next = newNode; // Link the new node at the end }
- Displaying the List: This function will help us see all the data in our list.
void display(Node* head) { while(head) { printf("%d -> ", head->data); head = head->next; // Move to the next node } printf("NULL\n"); }
- Main Function: Time to deine the main function and call above methods to create a singly linked list.
#include
#include # Add your functions here int main() { Node* head = NULL; // Initially, the list is empty append(&head, 10); // Adding 10 to our list append(&head, 20); // Adding 20 to our list append(&head, 30); // Adding 30 to our list display(head); // Should display: 10 -> 20 -> 30 -> NULL return 0; }
Wrapping Up
Creating and using a singly linked-list in C doesn’t have to be daunting. Think of it as a chain of boxes with each box pointing to the next. By understanding the basic operations (like creating a node, adding to the list, and displaying the list), you can explore more complex operations like deleting a node, reversing the list, and more. Happy coding!