JavaScript is a very popular programming language used all over the world. One useful feature of JavaScript is that it can check if the Caps Lock key is on or off. This is very important for things like password inputs where the user might not know that their Caps Lock is on. In this article, we will learn how to check if the Caps Lock key is on or off using JavaScript.
What you will learn?
- Understanding the KeyboardEvent object in JavaScript
- Detecting the status of the Caps Lock key
The KeyboardEvent Object
To check if the Caps Lock key is on, we first need to understand the KeyboardEvent object. Whenever a user presses or releases a key, a KeyboardEvent is created. This event contains useful information about the key that was pressed, including if any special keys like Shift or Ctrl were pressed.
Caps Lock Detection
To check if the Caps Lock key is on, we can use the getModifierState() method on the KeyboardEvent object. This method returns true or false depending on whether a special key like Caps Lock is active. The method takes one argument, which is a string representing the special key.
Here’s a simple example of using getModifierState() to check the Caps Lock status:
document.addEventListener('keydown', function(event) {
var capsIsOn = event.getModifierState('CapsLock');
console.log('Caps Lock status: ', capsIsOn ? 'On' : 'Off');
});
In the example above, we add an event listener to the document that listens for the ‘keydown’ event. The function gets the event object and then calls event.getModifierState(‘CapsLock’). This will return true if the Caps Lock key is on and false if it is off.
Implementing Visual Indicators for Caps Lock
It is very helpful to show a warning message when the Caps Lock key is on, especially during password input. Let’s create a function that shows a warning message when Caps Lock is on:
document.getElementById('password').addEventListener('keyup', function(event) {
var capsLockOn = event.getModifierState('CapsLock');
var warningElement = document.getElementById('caps-warning');
if (capsLockOn) {
warningElement.style.display = 'block';
} else {
warningElement.style.display = 'none';
}
});
In this code, we have an input field with the ID ‘password’ and a warning message with the ID ‘caps-warning’. When the Caps Lock key is on, the warning message is shown. When the Caps Lock key is off, the warning message is hidden.
Create an HTML Page
Here’s an HTML demo that includes an input field for a password and a warning message that appears when the Caps Lock key is on. You can also view this demo here:
<!DOCTYPE html>
<html>
<head>
<title>Detect Caps Lock in JavaScript</title>
<style>
#caps-warning {
display: none;
color: red;
}
</style>
</head>
<body>
<h1>Caps Lock Detection in JavaScript</h1>
<form>
<label for="password">Enter your password:</label><br>
<input type="password" id="password" name="password"><br>
<p id="caps-warning">Warning: Caps Lock is on!</p>
</form>
<script>
document.getElementById('password').addEventListener('keyup', function(event) {
var capsLockOn = event.getModifierState('CapsLock');
var warningElement = document.getElementById('caps-warning');
if (capsLockOn) {
warningElement.style.display = 'block';
} else {
warningElement.style.display = 'none';
}
});
</script>
</body>
</html>
In this demo, the warning message is hidden by default using CSS (display: none). The script listens for the ‘keyup’ event on the password input field. If the Caps Lock key is on, it changes the CSS of the warning message to display: block, showing the warning message. If the Caps Lock key is off, it hides the warning message by setting display: none.
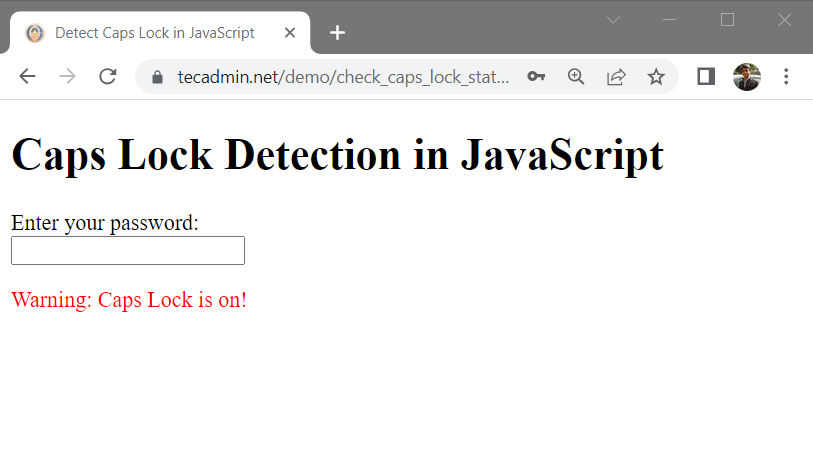
Points to Remember
This method only checks if the Caps Lock key is on at the time a key is pressed. It does not continuously monitor the status of the key.
The Caps Lock status might be different on different devices and operating systems. Always make sure your code works on different systems.
Conclusion
Checking if the Caps Lock key is on using JavaScript might seem hard at first, but it is easy once you understand the KeyboardEvent object and its methods. Adding this feature can improve the user experience by providing useful feedback. Always test your code on different devices and operating systems to make sure it works well.