Java, being one of the most versatile programming languages, offers multiple ways to accomplish tasks. Calculating the area of a circle is one such elementary problem that can be solved using Java. In this article, we’ll discuss how to calculate the area of a circle using Java and will provide a simple program as an example.
Formula to Calculate Area of a Circle
The formula to calculate the area of a circle is:
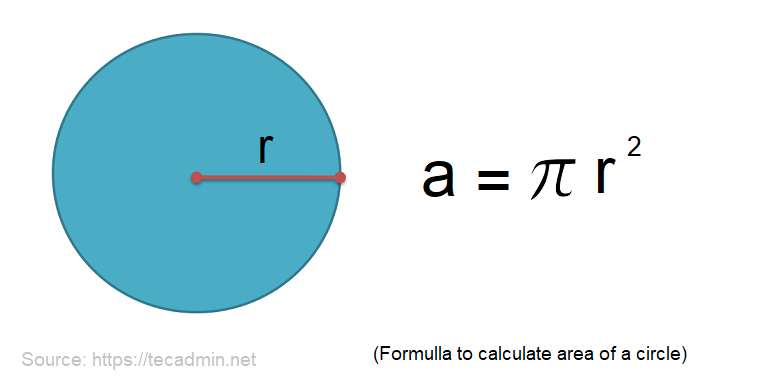
Where:
-
π
(Pi) is a constant approximately equal to 3.14159. -
r
is the radius of the circle.
Java Program Example
Lets check with an example of Java program that calculate area of a circle:
import java.util.Scanner;
public class CircleArea {
// Value of PI. We can also use Math.PI for a more accurate value.
private static final double PI = 3.14159;
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
// Prompt user for circle's radius
System.out.print("Enter the radius of the circle: ");
double radius = scanner.nextDouble();
// Calculate area
double area = PI * radius * radius;
// Display result
System.out.printf("The area of the circle with radius %.2f is %.2f", radius, area);
}
}
Explanation:
- Importing Scanner class: We import
java.util.Scanner
to read the radius value from the user. - Defining PI: We have defined a constant for the value of
π
(PI). However, Java’s Math library provides a more accurate value for PI, which can be accessed usingMath.PI
. - Taking Input: We use the Scanner class to read the radius of the circle. The
nextDouble()
method reads the radius as a double value.
Using Java’s Built-in PI Value
Java provides a built-in value for PI which is more precise. Here’s a modification of the above program using Math.PI:
import java.util.Scanner;
public class CircleArea {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter the radius of the circle: ");
double radius = scanner.nextDouble();
double area = Math.PI * radius * radius;
System.out.printf("The area of the circle with radius %.2f is %.2f", radius, area);
}
}
Compile and Run Above Java Programs
You can easily run this Java program on your own system. Just follow these steps:
- Save the Program: Copy the provided code and save it in a file named CircleArea.java.
- Compile the Program: Open a terminal or command prompt and navigate to the directory where you saved CircleArea.java. Compile the program by running the command:
javac CircleArea.java
This will create a CircleArea.class file in the same directory.
- Run the Program: Execute the program using the command:
java CircleArea
The program will prompt you to enter the radius of the circle. After you input a value, it will calculate and display the area of the circle.
Conclusion
Calculating the area of a circle is a fundamental operation, and Java provides a simple and straightforward way to accomplish this. Whether you’re using a constant value for PI or the built-in Math.PI, Java’s versatility ensures that you can calculate the circle’s area with precision.