Question: How do I remove the last character from a string in JavaScript or Node.js script?
This tutorial describes 2 methods to remove the last character from a string in JavaScript programming language. You can use any one of the following methods as per the requirements.
Method 1 – Using substring() function
Use the substring() function to remove the last character from a string in JavaScript. This function returns the part of the string between the start and end indexes, or to the end of the string.
Syntax:
1 | str.substring(0, str.length - 1); |
Example:
1 2 3 4 5 6 7 8 | // Initialize variable with string var str = "Hello TecAdmin!"; // Remove last character of string str = str.substring(0, str.length - 1); // Print result string console.log(str) |
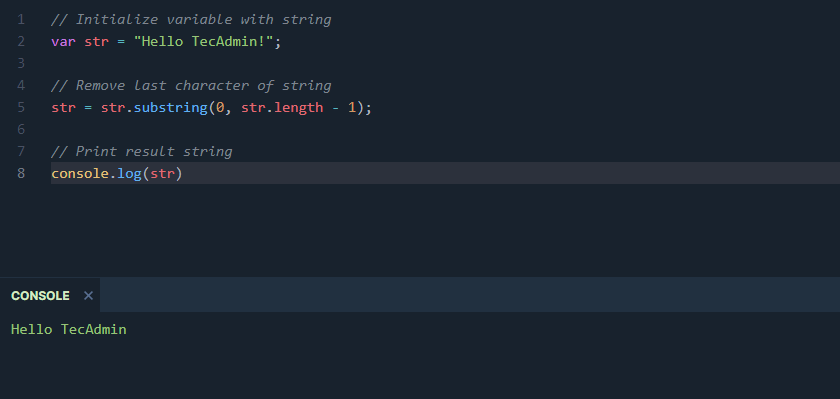
Method 2 – Using slice() function
Use the slice() function to remove the last character from any string in JavaScript. This function extracts a part of any string and return as new string. When you can store in a variable.
Syntax:
1 | str.slice(0, -1); |
Example:
1 2 3 4 5 6 7 8 | // Initialize variable with string var str = "Hello TecAdmin!"; // Remove last character of string str = str.slice(0, -1); // Print result string console.log(str) |
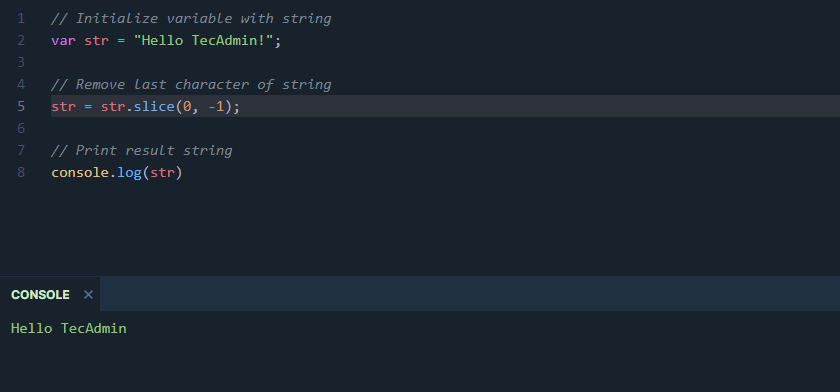
Wrap Up
In this tutorial, you have learned about removing the last character of a sting in JavaScript. We used substring() and slice() functions to alter a string.
3 Comments
if u want to cut a space char then use .trim()
Thanks
thanks